Editor’s Note: This article was written for older versions of GB Studio. As of GB Studio 3, you can now store 16 bit variables that range from -32,768 to 32,768. The techniques shown here are still useful for certain scenarios though.
One of the challenges creators face in GB Studio is the value limits on variables. With numbers that can only range from 0-255, this poses a problem for common game mechanics like Hi-Scores & Money systems. Sure, we could cap our wallets at 255 and call it day, but why stop there? We want 999 coins, not 255!
This article will cover how to do that, and we’ll be using everyone’s favorite tool: Math. We’ll cover how to create, display, and perform math on values of up to 999.
Part 0: Prep Work
To build a system for working with numbers above 255, you’ll want to be familiar with:
- Global Variables
- Event Groups
- The [Variable: Math Functions] event
- Compare Events (Variable to Value)
- Label: Define & Label: GoTo events
(Editor’s note: You can already find articles on this site for Variables, and there are ones upcoming for Math and Label events.)
Part 1: Breaking Down a Number
What you’ll need: 3 Global Variables.
To work with numbers above 255, we’ll be using variables to break down our numbers into their Ones, Tens, and Hundreds values. By storing a number’s components individually, we can “trick” the 255 limit. For example, the value of 999 can be thought of as:
- Hundreds = 9
- Tens = 9
- Ones = 9
These are actually 3 separate numbers – the only thing tying them together as ‘999’ is our understanding. If a player picks up a coin or scores a point, we’ll need to tell the system how to treat these numbers.
Let’s say we’re building a coin system. To start working these variables, first create 3 Global Variables, named “Coins – Ones”, “Coins – Tens”, and “Coins – Hundreds”. We’ll also need a way for our play to receive the coin, so we’ll create a treasure chest to award the player with some treasure.
In our actor, we’ll use a [Variable: Math] event to give the player 1 coin by selecting our “Coins – Ones” variable, and then adding a value of 1. After this event, let’s add a [Display Dialogue] event that shows how many coins we’re holding (you can display a variable by typing $$).
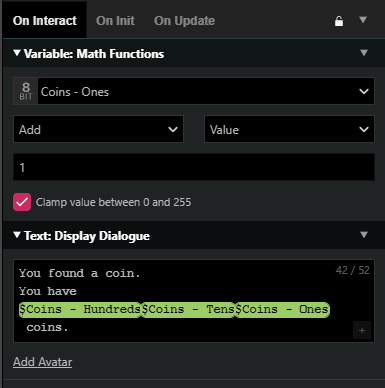
Now, whenever we interact with the treasure chest, our coins go up by 1, but is still acting as a standard use for a variable; it’s limited to 0-255, and will continue counting once we get our 10th coin or more. We’ll now need to build in some math events to move our money between our variables.
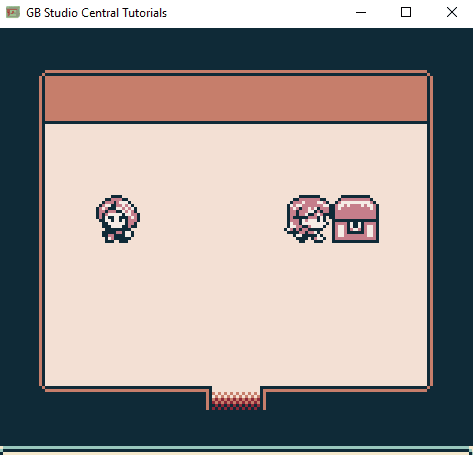
Part 2: Checking and Moving Between Variables
What you’ll need: Math Functions, Compare Events
When we receive our 10th coin, we want the value to display in our “Tens” variable, but we need to manually tell GB Studio to assign this. To do that, we’ll convert our Ones to Tens using Math.
In our treasure chest script, after the player receives their coin, we’ll want to check the current value of their “Ones”: if we have more than 10, we need to increase our “Tens” value. We can accomplish this with a Compare Variable to Value event.
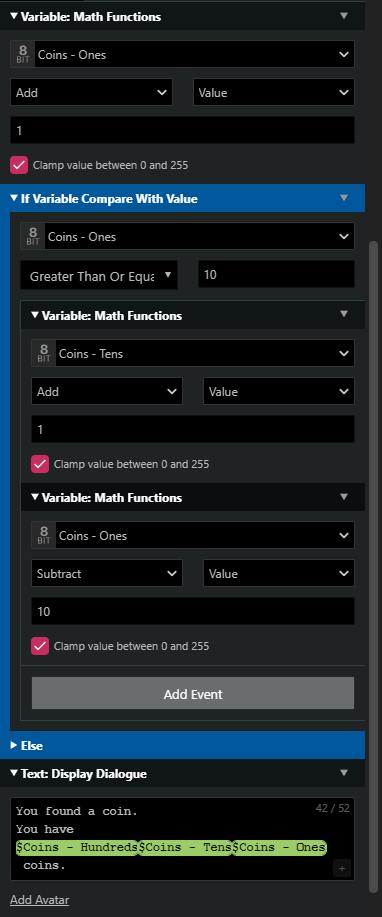
Now, when the player receives their coin, the script checks if we’ve gone above 10. If we’ve reached 10 or more, we increase “Tens” by 1, but we’ll also decrease “Ones” by 10. This builds in a range of 0-10 for our Ones value, and ensures that we are not just increasing both variables – we are essentially shifting 10 coins from one column to another. We can apply the same principle to our Tens and Hundreds by building in a second check: If Tens is ever greater than or equal to 10, we’ll subtract 10 from the Tens column, and increase our Hundreds by 1.
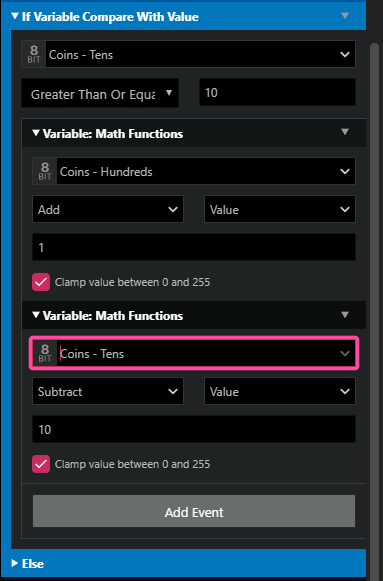
Finally, to create a cap, we’ll use another Compare Variable event. If our Hundreds value is greater than 9, we will use math to set all our variables to 9, creating a limit. You could go as far as you want with this, but we’ll be stopping at 999 for this tutorial.
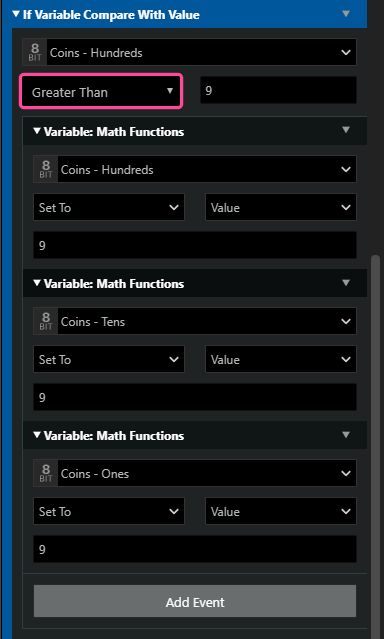
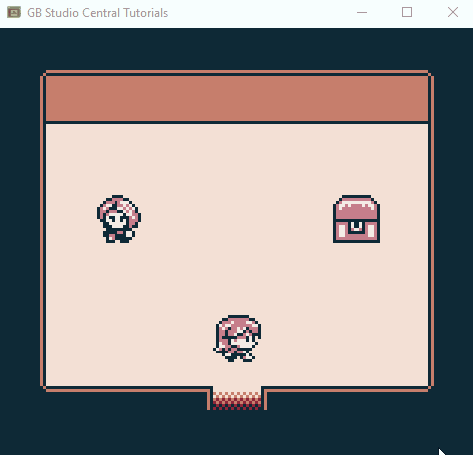
We can now carry up to 999 coins – and you can go further with more variables and math.
You can also change how the player is receiving coins: if you want the player to receive 200 coins, simplay add 2 to the Hundreds variable instead of 200 Ones.
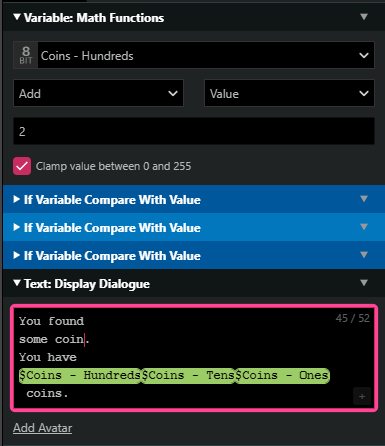
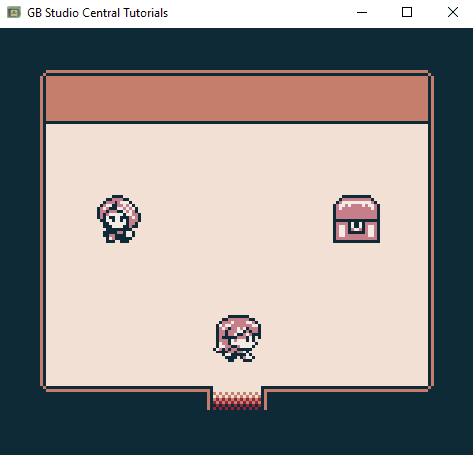
But, what if we did add 200 to our Ones variable? This system doesn’t account for that – yet. Let’s make that possible.
Part 3: Looping Our Math
What you’ll need: Event Groups, Label:Define, Label:Goto
Currently, our math system only checks our limits once, but we can create a loop to manage our money instantly. To make this easier, after we award our player with treasure, let’s organize our script into 3 event groups:
Event Group 1: Ones
Event Group 2: Tens
Event Group 3: Hundreds
In each event group, add a [Label: Define] event. This will assign a name to these sections so that we can return to them with the [Label: GoTo] event. Next, add [Compare Variable] events into their proper sections: Compare Variable (Ones) to the Ones Event group, Compare Variable (Tens) to the Tens event group and so on. You’ll want to make sure that each Compare Event is placed after the Label, so that we can return to the label without getting stuck in an infinite loop.
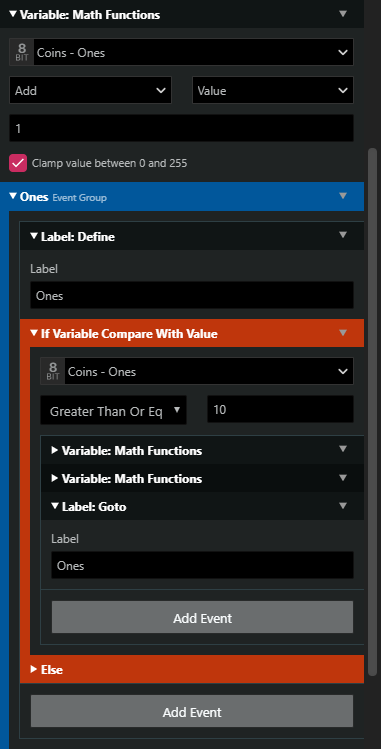
Within our Compare (Ones) event, after our math functions, place a Label:GoTo event to return to the Ones event group. Then, we’ll do the same for our Tens (returning to Tens).
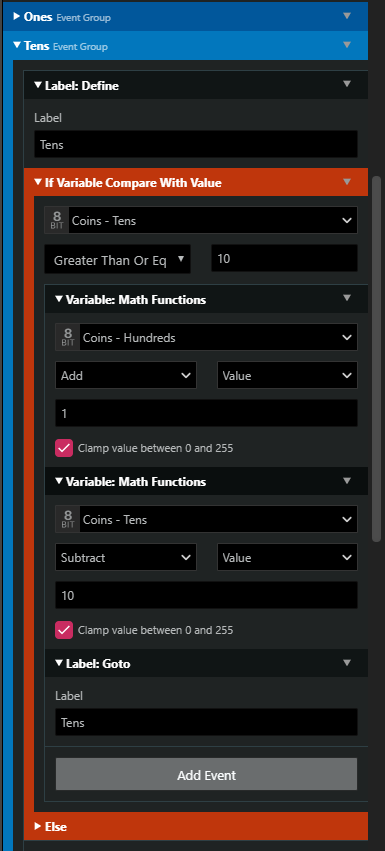
With this setup, when the player receives a coin, our script will check if the Ones value is greater than or equal to 10. If it is, it will increase the Tens value by 1 and subtract 10 from the Ones value, and then will return to the start of our math script to check again. If the Ones value is still greater than or equal to 10, it will repeat, and so on until we have a value of 0-9. When the Ones value is finally below 10, the script will proceed to the next event group: Tens. This will run a similar function: it will check our Tens value until it has reached a value of 0-9, increasing the Hundreds each time, then proceeding once complete.
Finally, in the Hundreds event group, place a Compare (Hundreds) event. Don’t use a Label: GoTo here, since we don’t repeat this step after it’s run.
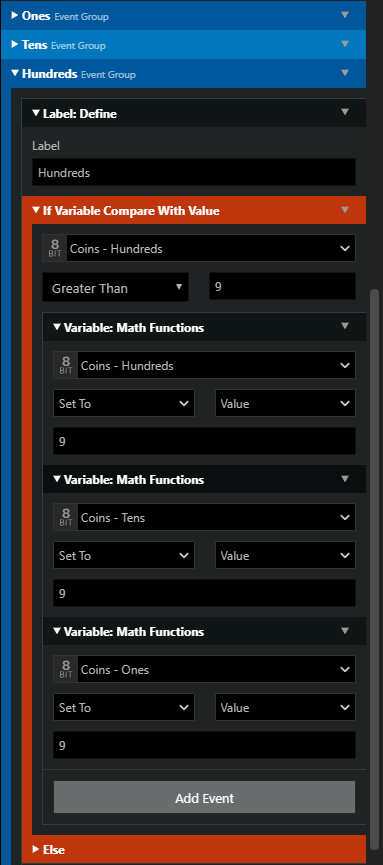
To see this in action, you can change the value of the Coins that the treasure chest rewards. For example, setting the treasure chest to Add 99 to the player’s Ones variable should result in the following:
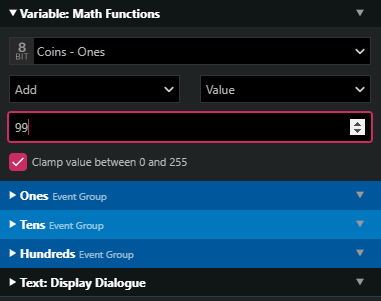
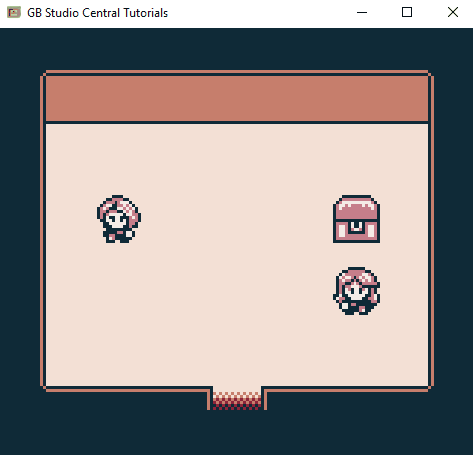
We’ve now successfully allowed the player to carry up to 999 coins. But what about when we want to spend them?
Part 4: Subtraction Across 3 Variables
What You’ll Need: Math Functions, Compare Variable to Variable/Value, Labels
What’s the point of having coins if we can’t spend them? Addition across 3 or more variables is one thing, but subtraction can be a little more complicated. For example, the player may have 10 coins, which is split between 1 Tens and 0 Ones. If we want there to be an action to spend 5 coins, a simple compare variable event would not work. It costs 5, but we have 0 ones. We need to build a way to move our money around to the other variables.
First, let’s create another actor to run the spending scripts, an NPC that will ask the player for 5 coins. In the Interact script, create a Compare Variable (Ones) to Value (5). In this event, if the Player has 5 or more coins in their Ones Variable, we’ll use Variable Math to Subtract 5 coins from Ones. If they don’t, the “Else” section will Display Dialogue: “You don’t have enough money”. You’ll want the player to have some money, so change the treasure chest to give the player 10 coins.
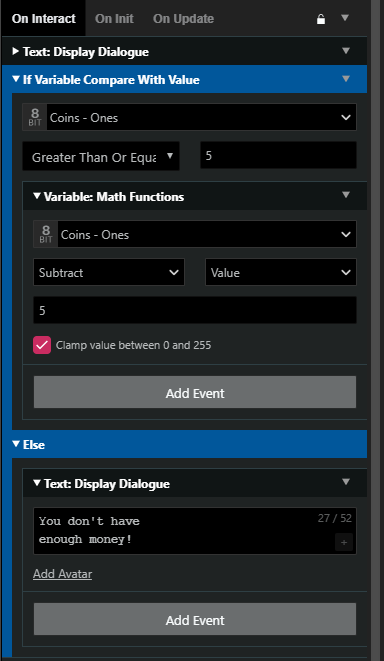
After we collect our coins, our “Ones” variable will be 0, so this is technically working, but we know we have 10 coins, the game doesn’t.
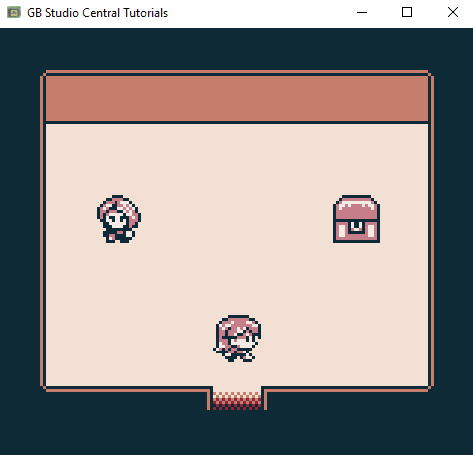
Just like with addition, we’ll need to build in loops to move our money between variables.
Back in “spend” actor, you’ll need to create more label loops. Before the Compare Variable event, put a new label: Price Check. This will allow for returning to the compare event to see if price is affordable yet.
Next, in the Compare Variable event, remove the Dialogue. Instead of simply stating that we can’t afford the price, it will now check to see if a Tens variable can be broken into Ones.
In the Else, create a new compare variable event to see if there are more than 0 tens. If the Tens value is greater than 0, subtract 1, and then add 10 to the Ones. Then, use a Label: GoTo to return to Price Check and check if it can be affordable again. Let’s also add a Display Dialogue event after we subtract to 5 coins to display the variables and check our work.
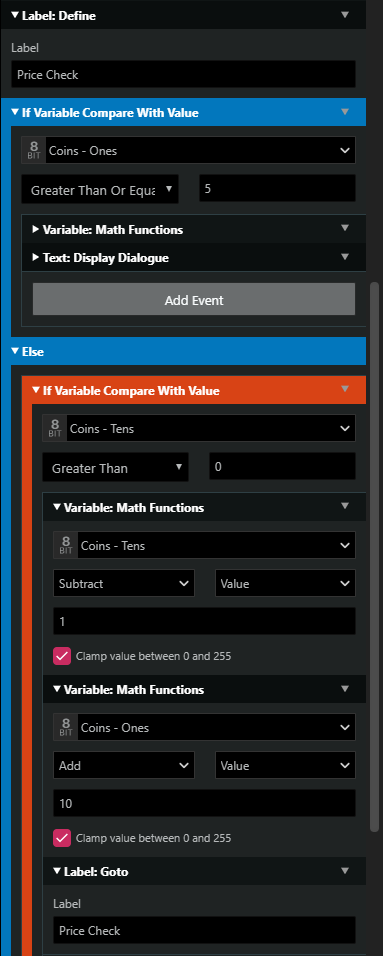
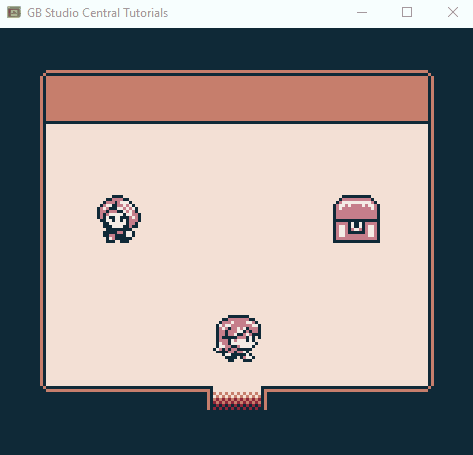
Now, instead of not recognizing that the player has enough coins, they can successfully give the NPC money two times.
We can also take this further – what if we had 100 coins? The Tens and the Ones would be checked as 0, but we know we can afford this. Just like with Tens to Ones, we can add a Hundreds to Tens check in our script.
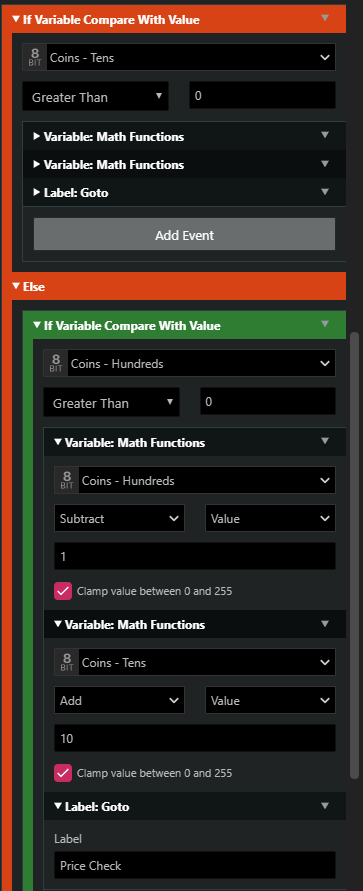
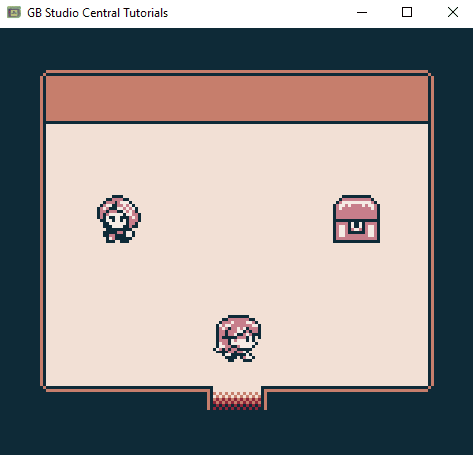
Wrapping Up: Managing Money
You now know how to manage your numbers across 3 variables with addition and subtraction. You may have noticed that these scripts can get quite long, especially if you repeat them to balance your variables after multiple rounds of addition and subtraction, or have gone beyond a Hundreds column.
In the next series, we will cover how to consolidate these scripts using Custom Events, as well as how to display your values on screen as well.
Tips and Tricks:
Don’t forget to clamp your variables! This is done via the “Clamp Variable” checkbox in Math Functions in 2.0, but will require manual checks in earlier versions.
As you test your script, insert Dialogue events to check your variable values and confirm your math.
If you’re moving values between variables often, you may end up with a Ones variable above 10. After running subtraction events, you can re-run the same events from gaining value to return each Ones/Tens/Hundreds column to their limits.
GB Studio 3.0 Note:
The Label and GoTo events have been deprecated. To create a label, use the GBVM Script event and simply type out a label name with underscores instead of spaces, and end it with a colon:
label_name:
To create GoTo event, use another GBVM Script event, using the term “VM_JUMP” followed by the label name:
VM_JUMP label_name
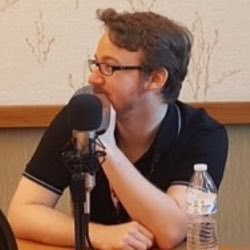
Friendly Neighborhood Game Designer, Podcaster, and (ex)Librarian (he/him)
GB Studio Games | Tabletop Games